WordPress Hacks and Tips #2
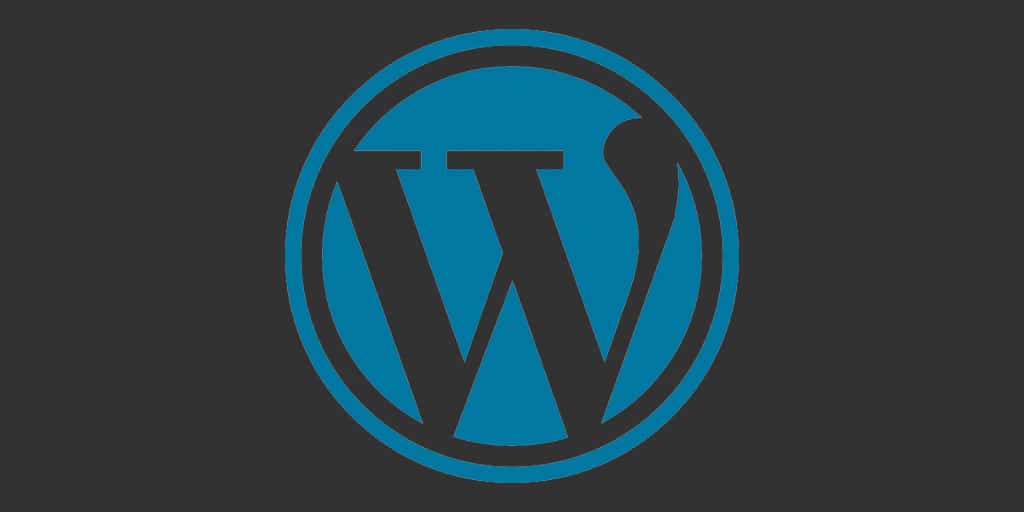
All of these hacks involve you adding code to your WordPress theme's functions.php file. If you don't know what or where that is, then it's probably best to avoid these tips for the time being. Bear in mind that if you are using an "off the shelf" theme, these settings may be overwritten when your theme is next updated. This isn't a problem if you are using your own custom theme or you are using a child theme.
Without further ado, let's get our hands dirty...
Change Howdy Greeting
It's easy to forget that WordPress was just a blogging platform now that it has evolved into a hugely powerful content management system. As I have been building a few large websites for businesses recently, I wanted to ensure a professional feel throughout- and that just had to mean that "Howdy, ..." greeting to change.
There are quite a few examples on the internet which try and implement this. Unfortunately, in my experience they didn't work with recent versions of WordPress, that is until I came across this code snippet from Greg Kerstin which does the job! I came across this on the WP Beginner site. Just paste the following code into your WordPress theme's functions.php file:
// Change Howdy add_action( 'admin_bar_menu', 'wp_admin_bar_my_custom_account_menu', 11 ); function wp_admin_bar_my_custom_account_menu( $wp_admin_bar ) { $user_id = get_current_user_id(); $current_user = wp_get_current_user(); $profile_url = get_edit_profile_url( $user_id ); if ( 0 != $user_id ) { /* Add the "My Account" menu */ $avatar = get_avatar( $user_id, 28 ); $howdy = sprintf( __('Welcome, %1$s'), $current_user->display_name ); $class = empty( $avatar ) ? '' : 'with-avatar'; $wp_admin_bar->add_menu( array( 'id' => 'my-account', 'parent' => 'top-secondary', 'title' => $howdy . $avatar, 'href' => $profile_url, 'meta' => array('class' => $class), )); } }
Migrate a Static website to WordPress
I've had to migrate quite a few old static websites to WordPress recently. Once I've built the WordPress website on a development server, I will want to migrate it over to the main website's domain. The problem with this, is that there is already a website there! How do I continue to serve the current static site to visitors, but let certain people through to see the WordPress website? Additionally, how do you then make the site live automatically on the live date? And how do I avoid having to alter any of the urls so that SEO won't be affected?
The good news, I've come up with a really good way of achieving all of this (helped by a rather splendid StackOverflow user, Jon Lin in this post) I've seen quite a few examples that rely on IP addresses to block or let visitors through. The problem is that many people have dynamic IP addresses that change regularly. This concept uses cookies to see if a user is "logged in".
First of all, you need to move your old site to a new directory. I'd recommend to /old/.
Then create a simple login page login.php in this folder. This sets a "login" cookie which will allow admins through:
<?php setcookie("login", "loggedin", time()+3600,"/"); header('Expires: Sat, 26 Jul 1997 05:00:00 GMT'); header('Cache-Control: no-store, no-cache, must-revalidate'); header('Cache-Control: pre-check=0, post-check=0, max-age=0'); ?> <h3>Welcome, you are logged in</h3> <p><a href="https://iag.me/">Click here to visit the home page</a></p>
Note that anyone coming to this login page will be given access to the WordPress website. You could always make it more secure by requiring a password. In most cases, the above solution should suffice, unless you want to protect a really top secret website!
You now can install your WordPress website. Because your old website is in the /old/ folder, you won't be overwriting anything. Actually migrating your WordPress from your development server to it's new home isn't something that I will be covering here. There are a number of tools that will help you here, such as Backup Buddy.
Once you've done this, head on down to your WordPress website's .htaccess file and add the following lines (before the WordPress rewrite bit)
RewriteCond %{HTTP_COOKIE} !^.*login.*$ [NC] RewriteCond %{TIME} <20120720200000 RewriteCond %{REQUEST_URI} !^/old/ RewriteRule (.*) /old/$1 [L]
The first line checks to see if the login cookie exists. If it doesn't and the time and date are before July 20, 2012 at 8pm (on the 2nd line), then rewrite each request to the /old/ folder unless you are already in the /old/ folder (the 3rd and 4th lines). If the visitor is logged in (i.e. the cookie is set) or is viewing the site after the live date, then do nothing... just let the WordPress rewrite rules do their job and server the WordPress website. Obviously, you'll have to change the date and time to the live date of your website. It is the year first, then the month, then the day and then the time- YYYYmmddHHmmss
Just one more thing, we have to get the WordPress rewrite rule to ignore everything in the /old/ folder. To do this we add a rule to the WordPress rewrite rules. You will need to add RewriteCond %{REQUEST_URI} !^/(old/.*)$
just before the first REQUEST_FILENAME
rule:
# BEGIN WordPress RewriteEngine On RewriteBase / RewriteRule ^index\.php$ - [L] RewriteCond %{REQUEST_URI} !^/(old/.*)$ RewriteCond %{REQUEST_FILENAME} !-f RewriteCond %{REQUEST_FILENAME} !-d RewriteRule . /index.php [L] # END WordPress
You then could set a countdown timer to countdown to the live date at which point the new WordPress website will appear as if by magic!
Enable HTTPS for logged in users and for certain pages
It's important to secure your WordPress website. This means adding an SSL certificate and redirecting users to the secure version of the website when logging in, accessing the admin area of the website and for any contact forms where they could be transmitting sensitive date.
The WordPress HTTPS plugin might work for most people, but it didn't seem to work for me and I quite liked having control over it myself.
I wanted a solution that redirected visitors to the secure HTTPS site when logged in, and also to redirect other visitors (who were not logged in) to the HTTPS site when viewing certain pages such as the contact and login pages.
The following code (which you add to your functions.php file) will:
- Redirect logged in users to the HTTPS site
- Redirect not logged in users to the HTTP site except...
- Redirect not logged in users visiting a contact or login page to the HTTPS site
$https_URL = "https://" . $_SERVER["SERVER_NAME"] . $_SERVER["REQUEST_URI"]; $http_URL = "http://" . $_SERVER["SERVER_NAME"] . $_SERVER["REQUEST_URI"]; if((strpos($_SERVER["REQUEST_URI"],"/login/") !== FALSE OR strpos($_SERVER["REQUEST_URI"],"/contact/") !== FALSE) && $_SERVER["HTTPS"] != "on") { header("Location: $https_URL",TRUE,301); exit(); } // This forces logged in users to use HTTPS if (is_user_logged_in() && $_SERVER["HTTPS"] != "on"){ $newurl = "https://" . $_SERVER["SERVER_NAME"] . $_SERVER["REQUEST_URI"]; header("Location: $https_URL"); exit(); } // Forces not logged in users to use HTTPS (except for certain pages) if (!is_user_logged_in() && $_SERVER["HTTPS"] == "on" && strpos($_SERVER["REQUEST_URI"],"/login/") === FALSE && strpos($_SERVER["REQUEST_URI"],"/contact/") === FALSE){ header("Location: $http_URL",TRUE,301); exit(); }
Customise Admin footer
Again, I've wanted to customise the dashboard part of a WordPress website by changing the footer to one of my choosing (perhaps a copyright notice). This hack is incredibly easy- just add the following code to your functions.php file (changing the text to whatever you want):
function remove_footer_admin () { echo '© 2012 - <a href="http://selectperformers.com/" title="Select Performers" target="_blank">Select Performers</a>'; } add_filter('admin_footer_text', 'remove_footer_admin');
Remove/add admin menu items for Editors
We at Select Performers tend to manage our clients' websites after launch. For this we take care of the WordPress theme and plugins as well as keeping them up to date. We give our clients access to their site- giving them the Editor role. This isn't a perfect solution as the Editor role misses some key features that we want to give our clients.
This isn't a big problem, as we can add certain features to the Editor role by adding code to the functions.php file.
Editor Access to Menu and Widgets (under "Appearance")
// get the the role object $role_object = get_role( 'editor' ); // add $cap capability to this role object $role_object->add_cap( 'edit_theme_options' );
This lets the Editor access the menu and widgets sections of the WordPress dashboard- useful if you want to give editors the ability to add or remove menu items and add widgets. It doesn't let the Editor add or remove themes, something which in my view is a good thing.
Editor Access to Viewing Gravity Forms Entries
I'm a big fan of Gravity Forms- it's so easy to use and is awesomely powerful at the same time. By adding the following code to the functions.php file, you can give your Editors the ability to view contact form entries but not be able to change the settings:
$editor = get_role( 'editor' ); $editor->remove_cap( 'gravityforms_edit_forms' ); $editor->remove_cap( 'gravityforms_delete_forms' ); $editor->remove_cap( 'gravityforms_create_form' ); $editor->add_cap( 'gravityforms_view_entries' ); $editor->add_cap( 'gravityforms_edit_entries' ); $editor->add_cap( 'gravityforms_delete_entries' ); $editor->remove_cap( 'gravityforms_view_settings' ); $editor->remove_cap( 'gravityforms_edit_settings' ); $editor->add_cap( 'gravityforms_export_entries' ); $editor->add_cap( 'gravityforms_view_entry_notes' ); $editor->add_cap( 'gravityforms_edit_entry_notes' );
Remove Items from WordPress Dashboard Side Bar
To remove clutter and unwanted items from the dashboard side bar is a good idea. This can make the WordPress dashboard area less daunting for new users. I'd also recommend removing most of the WordPress dashboard widgets too- but that's for another post!
This piece of code removes media, links, appearance, tools, settings, comments and plugins on the side bar for non-administrators:
function remove_menus () { if(!current_user_can('administrator')) { global $menu; $restricted = array(__('Media'), __('Links'), __('Appearance'), __('Tools'), __('Settings'), __('Comments'), __('Plugins')); end ($menu); while (prev($menu)){ $value = explode(' ',$menu[key($menu)][0]); if(in_array($value[0] != NULL?$value[0]:"" , $restricted)){unset($menu[key($menu)]);} } } } add_action('admin_menu', 'remove_menus');
Comments